How to Send an SMS Message in Java
Published: Jul 18, 2023
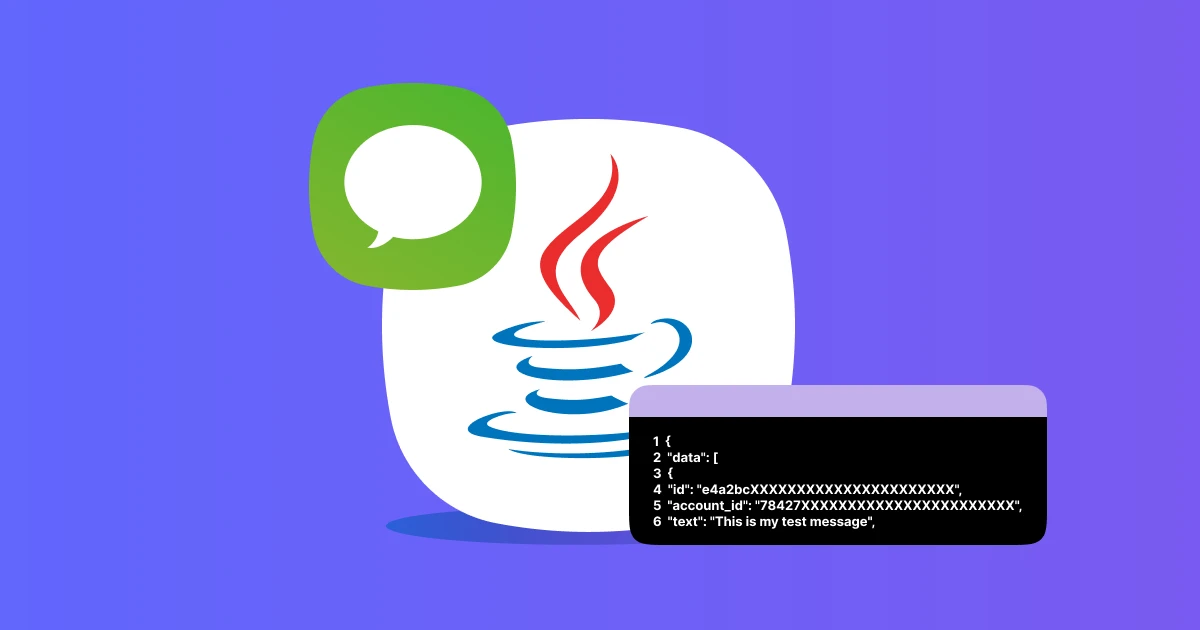
With SMS being one of the most effective marketing tools for businesses, making use of programmable texting services to deliver notifications has become a priority for developers. Using an SMS API service with a programming language like Java essentially allows you to integrate text messaging into your existing systems. Such services provide all the tools and resources a developer would need to send and receive SMS texts programmatically with Java. This article covers all you need to know about using Java to send SMS messages.
Why Use Java for SMS
Easy to Learn and Understand
Java is considered to be a simple programming language. It uses a syntax that’s rather straightforward to learn, write, maintain, and understand. The code is also easy to debug and is less complicated than other languages like C/C++. That is due to the removal of many complex features, such as storage classes and operator overloading, from Java.
Inexpensive to Maintain
Another upside of Java programs is that they are incredibly inexpensive to develop. They are also very economical to maintain. In other words, you can implement them on just about any machine, reducing the additional costs of maintenance in the process.
Supports Portability
Not being dependent on a specific platform makes Java a portable programming language. This means you can take it to any messaging platform you want and execute the code there. Needless to say, this portability is a great advantage for Java.
Object-Oriented
Java is an object-oriented programming language that can help make the code flexible and reusable. The concept of OOP makes it easy to reuse objects in other programs. It also enhances security by enabling you to bind the data and functions into one unit and make them inaccessible to the public. Moreover, it helps organize larger modules into smaller ones, making them easier to comprehend.
Platform-Independent
The Write Once Run Anywhere features of Java make it a platform-independent programming language. The language uses a compiled code (the byte code) that you can run on any machine regardless of the operating system—whether it’s Linux, Windows, or Mac OS.
Stable and Secure
In comparison to other languages, Java programs are more stable. And thanks to the removal of the explicit pointer concept, Java minimizes the risk of security breaches. It also offers a Security manager for every application, enabling you to define various access rules.
What You Need to Know Before Sending an SMS Via Java
To be able to send an SMS using Java, you’ll first need to choose a messaging provider that offers a Java API. Make sure to go through the provider’s API documentation to check if the solutions meet your business needs. After you’ve chosen your provider, you can initiate the integration process.
The first step is to ensure that you have a Java development environment (such as IntelliJ IDEA, Eclipse, Jetbrains Fleet, and Xcode) installed on your system. With many SMS providers, you’ll have the option to download their own Java library from GitHub. This makes the integration process easier.
Another prerequisite you need to account for is authorization. Different providers will have different methods of authorization, like Basic Auth, Bearer, Digest, and OAuth. You will also want to test the service by writing a Java code based on the documentation.
Get in Touch With Our Experts
Streamline your efforts and centralize your channels with Dexatel's all-in-one platform. Talk to our experts and get all your questions answered.
Coding for SMS involves using certain parameters that define the various elements of the text message you’re sending. These include the sender, the receiver, the text, and the communication channel.
The “from” parameter contains the sender ID you’ll be sending SMS messages from. It can contain up to 11 characters for an alphanumeric sender ID and up to 15 characters for phone numbers
The “to” parameter is the phone number (or numbers) to which you’ll send your SMS text message. Be sure that the phone numbers are in the international format with the destination country code
The “message” parameter consists of the message content that you want to send. This parameter can contain a maximum of 1,000 characters. Should the text be longer than 160 characters (70 for unicode), it will be divided into multiple messages
As for the “channel” parameter, it is optional and enables you to send the SMS message via the channel of your choice. You may switch to a different channel by simply updating the value in this parameter.
Sending an SMS Text Message With Java
To start sending SMS messages using Java, the first step is to open an account with a business messaging provider like Dexatel. You’ll also need an SMS-enabled phone number. Once you’ve signed up and purchased a number from the provider, simply follow the instructions below. Note that these steps demonstrate how to use Java to send a text message via Dexatel’s API.
Set up your Sender ID. This can be an alphanumeric text (your brand name, for example) or the mobile phone number from which you’re sending the SMS text
Create an API key and, using the Java Helper library, make an HTTP POST request to Dexatel Endpoints. Here’s an example of an SMS request:
Unirest.setTimeouts(0, 0);
HttpResponse<String> response = Unirest.post("https://api.dexatel.com/v1/messages")
.header("Content-Type", "application/json")
.header("X-Dexatel-Key", "0940dXXXXXXXXXXXXXXXXXX")
.body("{\n\"data\": {\n\"from\": \"Dexatel2\",\n\"to\": [\n\"18558675310\"\n],\n\"text\": \"This is my 6 test message\",\n\"channel\": \"sms\"\n}\n}")
.asString();
And this is an example of a Json response:
{
"data": [
{
"id": "e4a2bcXXXXXXXXXXXXXXXXXXXXXX",
"account_id": "78427XXXXXXXXXXXXXXXXXXXXXXX",
"text": "This is my test message",
"from": "Dexatel2",
"to": "18558675310",
"channel": "SMS",
"status": "enroute",
"create_date": "2023-06-12 12:00:42",
"update_date": "2023-06-12 12:00:42",
"encoding": "GSM-7",
"segment_count": 1
}
]
}
Receiving an SMS Text With Java
Communication is a two-way street, and sending a text message is only one-half of it. That being said, many messaging providers support the receipt of SMS texts. When a customer sends an SMS message, you can use a web app like Spark to receive it on your server.
To install Spark, you’ll want to edit pom.xml and add dependencies—as shown below—for both the web app as well as the Simple Logging Facade for Java (SLF4J)
<dependency>
<groupId>com.sparkjava</groupId>
<artifactId>spark-core</artifactId>
<version>2.9.1</version>
</dependency>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-simple</artifactId>
<version>1.7.21</version>
</dependency>
Create a Java class in the project with the name ReceiveSMS and enter this code into it:
import static spark.Spark.*;
public class ReceiveSms {
public static void main(String[] args) {
get("/receive_sms", (request, response) -> {
// Sender's phone number
String from_number = request.queryParams("From");
// Receiver's phone number - Plivo number
String to_number = request.queryParams("To");
// The text that was received
String text = request.queryParams("Text");
// Print the message
System.out.println(from_number + " " + to_number + " " + text);
return "Message Received";
});
}
}
The above method is great for testing. But if you want to connect to the internet in order to receive incoming messages as well as handle callbacks, you’ll need to use a program like ngrok. Once you download and run the program, specify the port hosting the application where you’ll be receiving the SMS messages. The command line should look like this:
./ngrok http [portnum]
Additional Features of Java SMS APIs
Monitoring the Status of Your Message
Once you send out a text message, you can keep track of its status to check if it successfully reaches its destination. Many SMS API providers offer webhooks or callback mechanisms that notify your application about the status of the messages you’ve sent. You can use these callbacks for updating your system’s database or activating certain actions, like a follow-up text.
Replying to an SMS Sent to Your Number
You can use the Java SMS API to configure a phone number to receive incoming text messages. Setting up a webhook or callback URL enables you to handle incoming messages and program replies to those messages. After you receive a text, your application will assess its content, carry out the necessary processing, and respond to it using the SMS API.
Managing Message State
Message state management is where you monitor the SMS text messages you send and the metadata associated with them. This can include timestamps, message IDs, message content, and recipient phone numbers. By storing this information in the database of your application, you can carry out actions such as generating reports, retrieving message history, and more.
API Reference Documentation for Messages
API reference documentation serves as a guide for developers on how to interact with the SMS API using Java. It does so by providing detailed information about the SMS API methods, parameters, and response formats. The documentation normally consists of code examples, details about available functions, and explanations of request and response structures.
High-Volume Messages
High-volume messaging is when you send a large number of SMS messages in a short period of time. SMS API providers often come in handy in this regard, by providing solutions like message queuing, message throttling, and advanced message routing mechanisms. API providers typically offer dedicated support and tailored solutions that serve high-volume messaging purposes.
SMS API Services
Twilio
As one of the leading cloud communications platforms, Twilio provides a comprehensive list of APIs for SMS, voice, and video messaging. Developers can use Twilio’s SMS API to send and receive text messages programmatically. The platform offers a number of features, like two-way texting, message status tracking, and multichannel support. Twilio’s reliability, scalability, and extensive documentation make it an excellent choice for developers.
Vonage API Platform
Formerly known as Nexmo, the Vonage API Platform offers a wide variety of APIs for texting, voice, and authentication. The platform’s SMS API allows developers to send text messages globally and supports Unicode messaging, delivery receipts, SMS concatenation, and opt-out handling. With extensive global coverage and support for multiple programming languages, the platform is accessible to developers worldwide.
MessageBird
Messagebird provides an SMS API that developers can use to send and receive messages worldwide with both long and short codes. Besides global coverage and high rates of delivery, the platform also offers support for various integrations and programming languages like Java. Other API features include message scheduling, templates, delivery reports, and phone number verification.
Dexatel
Dexatel offers an SMS API that allows for effortless automation of text messages. Developers can integrate Dexatel’s REST API to communicate with customers in the form of transactional as well as marketing messages. Each SMS text that you send through the API will have its own unique ID, enabling you to obtain information regarding the delivery status. The API also supports various features, including webhooks, templates, and verification.
When choosing an API provider, keep in mind that the setup processes vary from one platform to another. You may also want to account for various factors like pricing, documentation, scalability, and customer support, in addition to the specific needs of your application.
Key Takeaways
The importance of effective business messaging is driving developers to make use of Java to send SMS messages. The programming language is easy to get to grips with and offers advantages like portability and platform independence. SMS API providers such as Dexatel and Twilio enable developers to send and receive SMS messages using Java. And with the instructions you’ll find in this article, you can get started easily and in a matter of minutes.